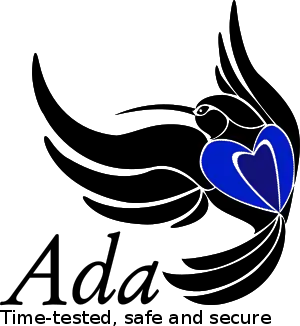
This language feature is only available from Ada 2005 on. Ada.Directories is a unit of the Predefined Language Environment since Ada 2005. It allows various manipulations and queries of the filesystem directories.
The Package Directories
Used for simple directory manipulation as defined in Ada 2005.
Traversing Directories
Changing the current working directory is done with Set_Directory
. The remaining functions are fairly self-explanatory with the exception of Containing_Directory
, which returns the name of the directory containing the given directory.
function
Current_Directoryreturn
String;procedure
Set_Directory (Directory :in
String);function
Exists (Name :in
String)return
Boolean;function
Containing_Directory (Name :in
String)return
String;
Enumerating Directory Entries
This example enumerates the all the entries in the current working directory, that end in ".gpr". The Start_Search
procedure is used to start enumerating a directory. It will To remove the filter criteria you can pass an empty string. Iterating through the directory involves calling Get_Next_Entry
to return the next directory entry and More_Entries
to check for more entries. Once the search is complete, call End_Search
.
type
Search_Typeis
limited
private
;type
Directory_Entry_Typeis
limited
private
;procedure
Start_Search (Search :in
out
Search_Type; Directory :in
String; Pattern :in
String; Filter :in
Filter_Type := (others
=> True));procedure
Get_Next_Entry (Search :in
out
Search_Type; Directory_Entry :out
Directory_Entry_Type);function
More_Entries (Search :in
Search_Type)return
Boolean;procedure
End_Search (Search :in
out
Search_Type);
The resulting Directory_Entry_Type has a full name, simple name, size, kind, and modification type.
function
Simple_Name (Directory_Entry :in
Directory_Entry_Type)return
String;function
Full_Name (Directory_Entry :in
Directory_Entry_Type)return
String;function
Kind (Directory_Entry :in
Directory_Entry_Type)return
File_Kind; -- Directory, Ordinary_File, Special_Filefunction
Size (Directory_Entry :in
Directory_Entry_Type)return
File_Size;function
Modification_Time (Directory_Entry :in
Directory_Entry_Type)return
Ada.Calendar.Time;
with
Ada.Text_IO;with
Ada.Directories;use
Ada.Text_IO;use
Ada.Directories;procedure
Mainis
Dir : Directory_Entry_Type; Dir_Search : Search_Type; Curr_Dir : string := Current_Directory;begin
Put("Current Directory: "); Put_Line(Curr_Dir); Start_Search(Search => Dir_Search, Directory => Curr_Dir, Pattern => "*.gpr");loop
Get_Next_Entry(Dir_Search, Dir); Put(Full_Name(Dir)); Set_Col(50);if
Kind(Dir) = Ordinary_Filethen
Put(Size(Dir)'Image);end
if
; Set_Col(60); Put_Line(Kind(Dir)'Image);exit
when
not
More_Entries(Dir_Search);end
loop
; End_Search(Dir_Search);end
Main;
Directory Manipulation
Creating a directory is done with Create_Directory
. Create_Path
creates all the directories in the path. The directory path on GNAT Ada can contain either "\" or "/" characters. Rename
renames a directory or file within a directory. <Delete_Tree> deletes an entire hierarchy of directories and files. Delete_Directory
deletes an empty directory (non-empty directories throw a Use_Error
exception. Delete_File
deletes an ordinary file in a directory. Note that the Form
parameters is implementation specific. On the version of GNAT Ada I'm using, it can only be used to set the encoding.
procedure
Create_Directory (New_Directory :in
String; Form :in
String := "");procedure
Create_Path (New_Directory :in
String; Form :in
String := "");procedure
Rename (Old_Name, New_Name :in
String);
procedure
Delete_Tree (Directory :in
String);procedure
Delete_Directory (Directory :in
String);procedure
Delete_File (Name :in
String);
Below is a simple example that creates a set of directories and iterates through those directories. Note that it runs on both Windows and Linux with the "/" path separator. The returned path separators are appropriate to the filesystem (e.g. Windows return "\").
procedure
Mainis
Dir : Directory_Entry_Type; Dir_Search : Search_Type;begin
Start_Search(Search => Dir_Search, Directory => Curr_Dir, Pattern => ""); Create_Path("Foo"); Create_Path("Bar/Baz/Qux");loop
Get_Next_Entry(Dir_Search, Dir); Put_Line(Full_Name(Dir));exit
when
not
More_Entries(Dir_Search);end
loop
; End_Search(Dir_Search); Delete_Directory("Foo"); Delete_Tree("Bar");end
Main;
Exceptions
Status_Error
is raised if a directory entry is invalid or when enumerating directories past the last directory. Name_Error
is usually thrown if the directory or filename is not able to identify a file or directory (either non-existent or invalid depending on context). Use_Error
is thrown if directories are not supported or directories are not traversable.
Status_Error :exception
renames
Ada.IO_Exceptions.Status_Error; Name_Error :exception
renames
Ada.IO_Exceptions.Name_Error; Use_Error :exception
renames
Ada.IO_Exceptions.Use_Error; Device_Error :exception
renames
Ada.IO_Exceptions.Device_Error;
Specification
-- Standard Ada library specification -- Copyright (c) 2003-2018 Maxim Reznik <reznikmm@gmail.com> -- Copyright (c) 2004-2016 AXE Consultants -- Copyright (c) 2004, 2005, 2006 Ada-Europe -- Copyright (c) 2000 The MITRE Corporation, Inc. -- Copyright (c) 1992, 1993, 1994, 1995 Intermetrics, Inc. -- SPDX-License-Identifier: BSD-3-Clause and LicenseRef-AdaReferenceManual -- -------------------------------------------------------------------------with
Ada.Calendar;with
Ada.IO_Exceptions;package
Ada.Directoriesis
-- Directory and file operations:function
Current_Directoryreturn
String;procedure
Set_Directory (Directory :in
String);procedure
Create_Directory (New_Directory :in
String; Form :in
String := "");procedure
Delete_Directory (Directory :in
String);procedure
Create_Path (New_Directory :in
String; Form :in
String := "");procedure
Delete_Tree (Directory :in
String);procedure
Delete_File (Name :in
String);procedure
Rename (Old_Name :in
String; New_Name :in
String);procedure
Copy_File (Source_Name :in
String; Target_Name :in
String; Form :in
String := ""); -- File and directory name operations:function
Full_Name (Name :in
String)return
String;function
Simple_Name (Name :in
String)return
String;function
Containing_Directory (Name :in
String)return
String;function
Extension (Name :in
String)return
String;function
Base_Name (Name :in
String)return
String;function
Compose (Containing_Directory :in
String := ""; Name :in
String; Extension :in
String := "")return
String; -- File and directory queries:type
File_Kindis
(Directory, Ordinary_File, Special_File);type
File_Sizeis
range
0 .. implementation_defined;function
Exists (Name :in
String)return
Boolean;function
Kind (Name :in
String)return
File_Kind;function
Size (Name :in
String)return
File_Size;function
Modification_Time (Name :in
String)return
Ada.Calendar.Time; -- Directory searching:type
Directory_Entry_Typeis
limited
private
;type
Filter_Typeis
array
(File_Kind)of
Boolean;type
Search_Typeis
limited
private
;procedure
Start_Search (Search :in
out
Search_Type; Directory :in
String; Pattern :in
String; Filter :in
Filter_Type := (others
=> True));procedure
End_Search (Search :in
out
Search_Type);function
More_Entries (Search :in
Search_Type)return
Boolean;procedure
Get_Next_Entry (Search :in
out
Search_Type; Directory_Entry :out
Directory_Entry_Type);procedure
Search (Directory :in
String; Pattern :in
String; Filter :in
Filter_Type := (others
=> True); Process :not
null
access
procedure
(Directory_Entry :in
Directory_Entry_Type)); -- Operations on Directory Entries:function
Simple_Name (Directory_Entry :in
Directory_Entry_Type)return
String;function
Full_Name (Directory_Entry :in
Directory_Entry_Type)return
String;function
Kind (Directory_Entry :in
Directory_Entry_Type)return
File_Kind;function
Size (Directory_Entry :in
Directory_Entry_Type)return
File_Size;function
Modification_Time (Directory_Entry :in
Directory_Entry_Type)return
Ada.Calendar.Time; Status_Error :exception
renames
Ada.IO_Exceptions.Status_Error; Name_Error :exception
renames
Ada.IO_Exceptions.Name_Error; Use_Error :exception
renames
Ada.IO_Exceptions.Use_Error; Device_Error :exception
renames
Ada.IO_Exceptions.Device_Error;private
pragma
Import (Ada, Directory_Entry_Type);pragma
Import (Ada, Search_Type);end
Ada.Directories;
See also
Wikibook
External examples
- Search for examples of
Ada.Directories
in: Rosetta Code, GitHub or this Wikibook. - Search for any post related to
Ada.Directories
in: Stack Overflow, comp.lang.ada or any Ada related page.
Tutorials
Ada Reference Manual
Ada 2005
Ada 2012
Open-Source Implementations
FSF GNAT
- Specification: a-direct.ads
- Body: a-direct.adb
drake
- Specification: directories/a-direct.ads
- Body: directories/a-direct.adb